JSON Response
Django에서는 JsonResponse 클래스를 사용해 간편하게 JSON 형태의 응답을 만들 수 있음
프로젝트 기본 설정
프로젝트 디렉토리 생성 및 초기 세팅
mkdir api_pjt
cd api_pjt
python -m venv .venv
pip install django
Django 프로젝트 시작
django-admin startproject api_pjt .
프로젝트 이름: api_pjt
앱 생성
python manage.py startapp articles
앱 이름: articles
settings.py 설정
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
# Third-party
"django_seed",
# Local
"articles",
]
모델 정의
articles/models.py
from django.db import models
class Article(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
created_at = models.DateTimeField(auto_now_add=True)
updated_at = models.DateTimeField(auto_now=True)
테스트 데이터 만들기 (Django Seed 사용)
GitHub - Brobin/django-seed: :seedling: Seed your Django database with fake data
:seedling: Seed your Django database with fake data - Brobin/django-seed
github.com
설치
pip install django-seed==0.2.2
pip freeze > requirements.txt
시드 데이터 생성
python manage.py seed articles --number=30
* psycopg2 관련 에러 발생 시:
pip install psycopg2
마이그레이션
python manage.py makemigrations
python manage.py migrate
URL 연결
api_pjt/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path("admin/", admin.site.urls),
path("api/v1/articles/", include("articles.urls")),
]
HTML Response (기존 방법)
URLConf
articles/urls.py
from django.urls import path
from . import views
app_name = "articles"
urlpatterns = [
path("html/", views.article_list_html, name="article_list_html"),
]
View 함수
articles/views.py
from django.shortcuts import render
from .models import Article
def article_list_html(request):
articles = Article.objects.all()
context = {"articles": articles}
return render(request, "articles/articles.html", context)
템플릿 파일
templates/articles/articles.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Article List</title>
</head>
<body>
<h1>Article List</h1>
<hr>
{% for article in articles %}
<h3>title: {{ article.title }}</h3>
<p>content: {{ article.content }}</p>
<p>created_at: {{ article.created_at }}</p>
<p>updated_at: {{ article.updated_at }}</p>
<hr>
{% endfor %}
</body>
</html>
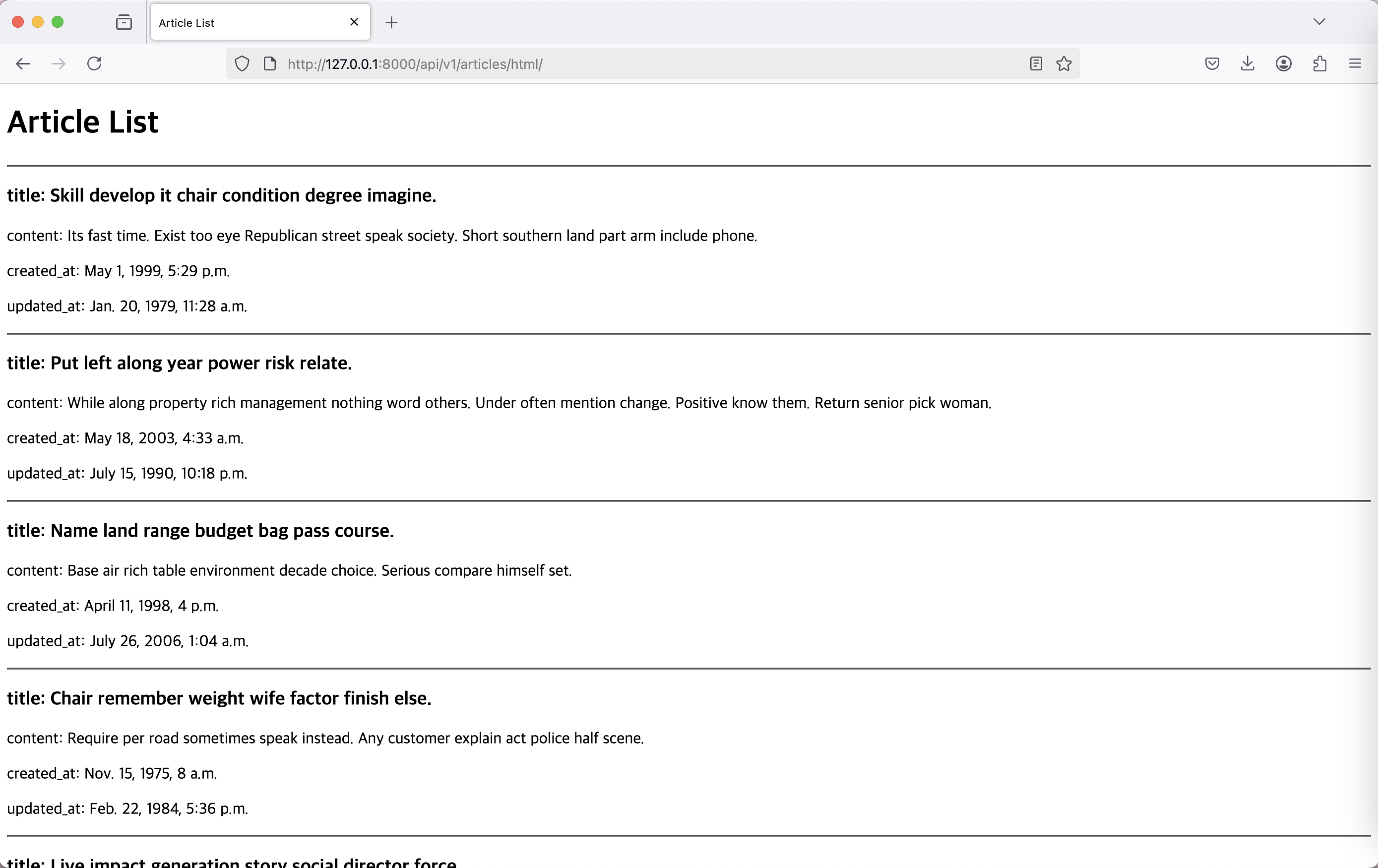
JSON Response - 방법 1 (직접 딕셔너리 구성)
URL 추가
articles/urls.py
path("json-01/", views.json_01, name="json_01"),
View 함수
articles/views.py
from django.http import JsonResponse
def json_01(request):
articles = Article.objects.all()
data = [
{
"title": article.title,
"content": article.content,
"created_at": article.created_at,
"updated_at": article.updated_at,
}
for article in articles
]
return JsonResponse(data, safe=False)
JsonResponse는 기본적으로 dict만 허용하지만, safe=False를 주면 list도 반환 가능
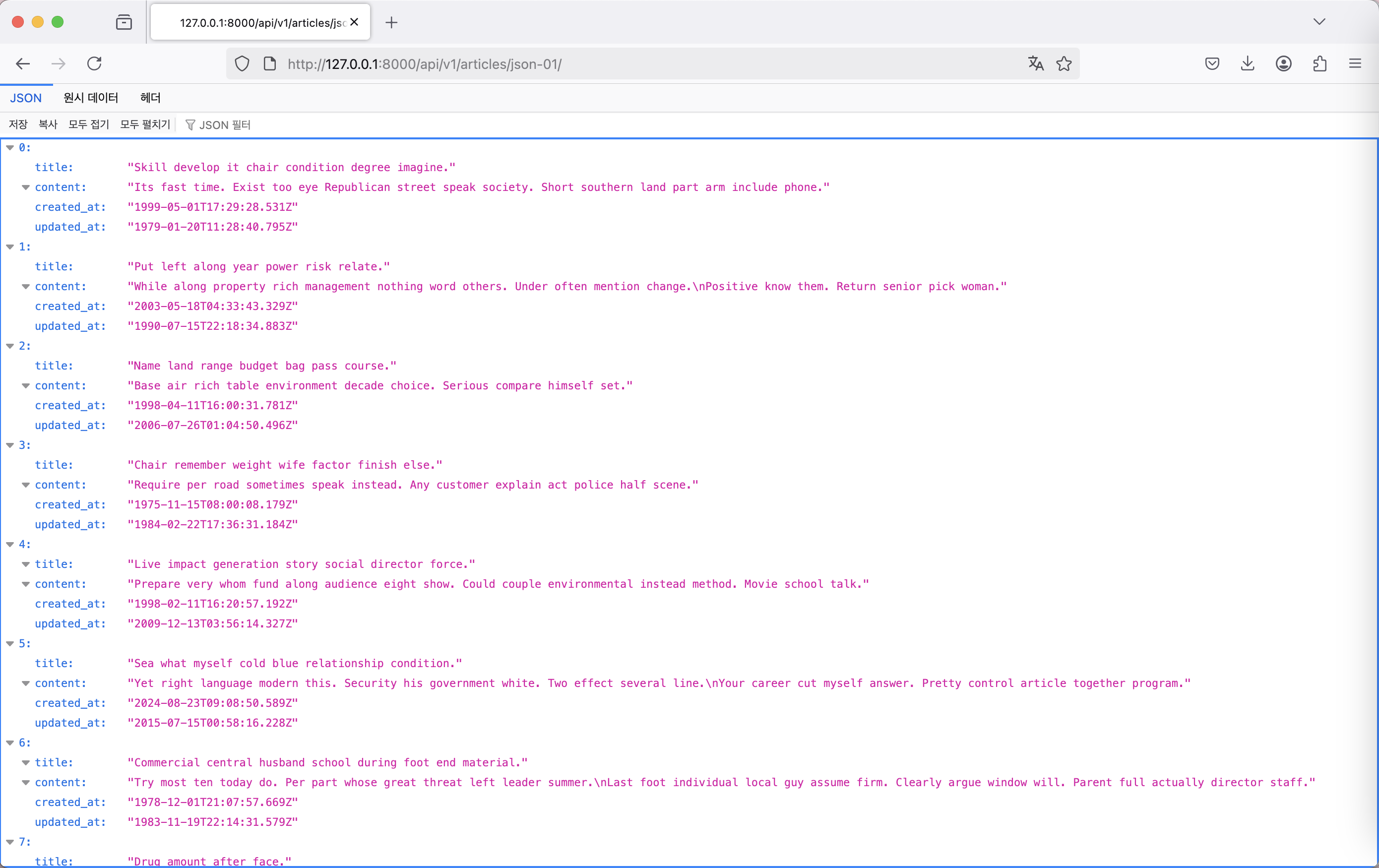
JSON Response - 방법 2 (Django Serializer 사용)
URL 추가
articles/urls.py
path("json-02/", views.json_02, name="json_02"),
View 함수
articles/views.py
from django.http import HttpResponse
from django.core import serializers
def json_02(request):
articles = Article.objects.all()
data = serializers.serialize("json", articles)
return HttpResponse(data, content_type="application/json")
serializers.serialize()는 모델 구조에 종속적인 기본 구조만 출력됨 → 유연성 떨어짐
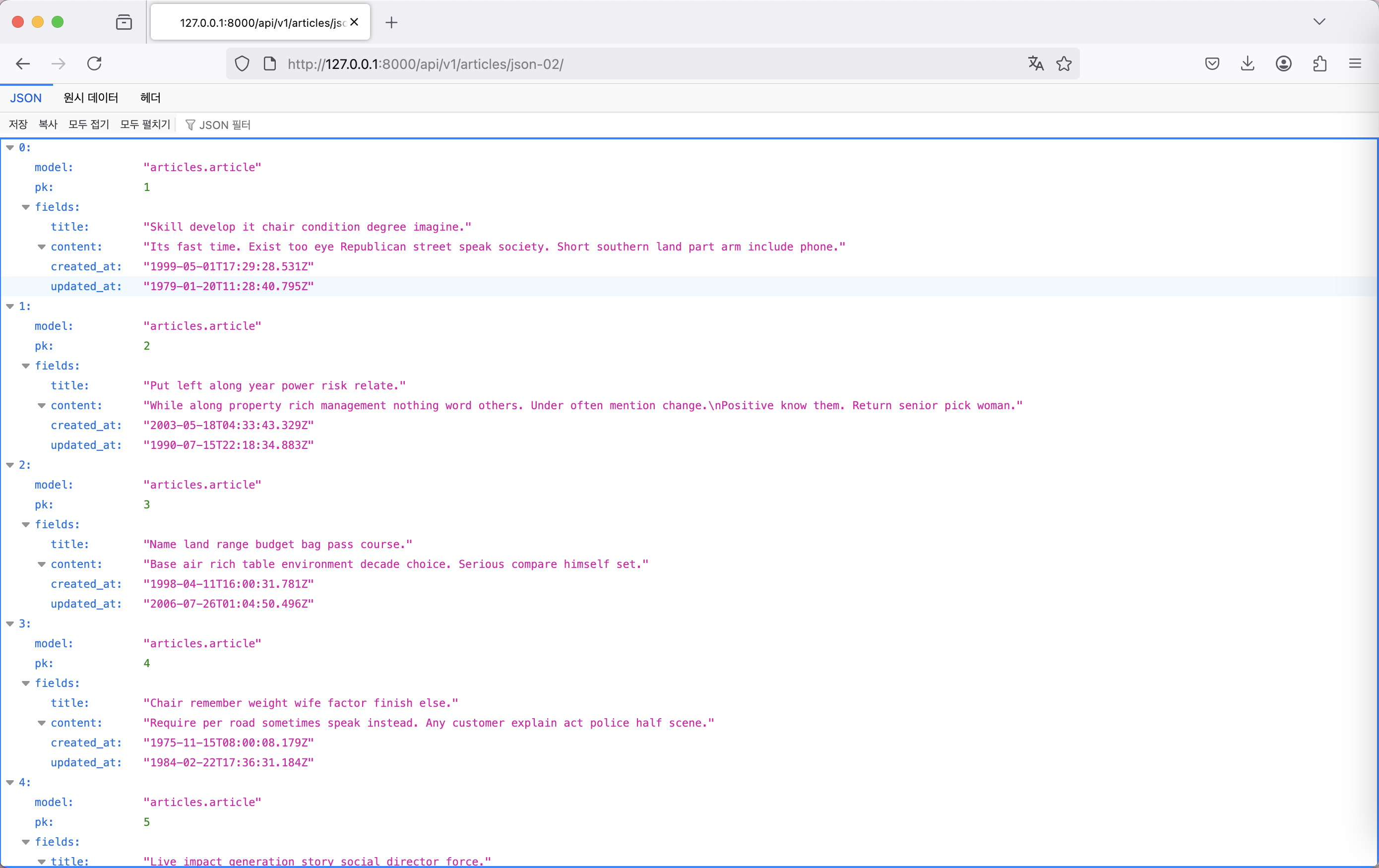
직렬화(Serialization)
Python 객체나 DB 모델을 전송하거나 저장할 수 있는 형태(JSON, XML 등)로 변환하는 과정
(데이터의 구조는 유지, 추후 재구성이 가능한 포맷으로 변환)
Django는 기본적으로 serializers.serialize() 방식 지원 (serializers 모듈) : Model → JSON 변환
모델 구조에 한정적이며 유연한 필드 구성, 커스텀 응답 구조에는 한계가 있음 (뷰 로직과 연결 어려움)
Django REST Framework(DRF)의 Serializer 클래스
- 모델에 의존하지 않거나 유연한 데이터 구조 정의 가능
- APIView, Response 등과 연동 쉬움
- 응답 구조를 쉽게 커스터마이징할 수 있어 REST API 설계에 적합
'∟ Framework > ∟ DRF' 카테고리의 다른 글
DRF Single Model CRUD API 구현 (0) | 2025.03.29 |
---|---|
Django REST Framework(DRF) API 구현 (0) | 2025.03.29 |
RESTful API와 JSON (1) | 2025.03.28 |
HTTP(Hyper Text Transfer Protocol)와 URL(Uniform Resource Locator) 구조 (0) | 2025.03.28 |
DRF(Django REST Framework) (1) | 2025.03.25 |